- Published on
go-zero集成consul
- Authors
- Name
- liuxiaobo
服务注册
goctl rpc new greet
修改配置
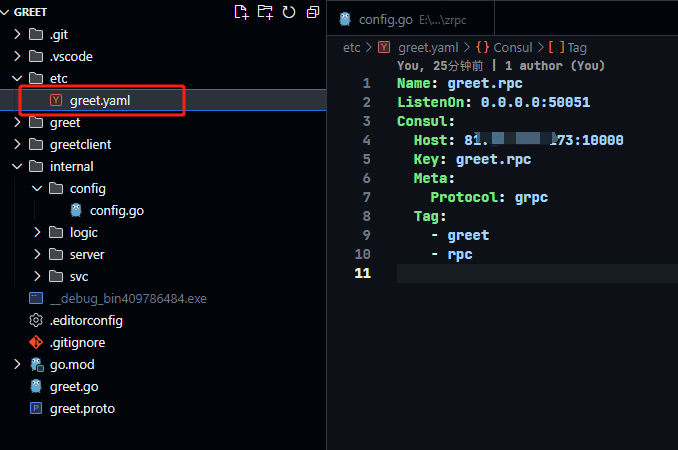
Consul:
Host: x.x.x.x:10000
Key: greet.rpc
Meta:
Protocol: grpc
Tag:
- greet
- rpc

package config
import (
"github.com/zeromicro/go-zero/zrpc"
"github.com/zeromicro/zero-contrib/zrpc/registry/consul"
)
type Config struct {
zrpc.RpcServerConf
Consul consul.Conf
}
注册到consul

"github.com/zeromicro/zero-contrib/zrpc/registry/consul"
if err := consul.RegisterService(c.ListenOn, c.Consul); err != nil {
panic(err)
}
效果

服务发现
goctl api new greet_client
修改配置

Name: greet_client-api
Host: 0.0.0.0
Port: 20000
GreetRpc:
Target: consul://x.x.x.x:10000/greet.rpc?wait=14s

package config
import (
"github.com/zeromicro/go-zero/rest"
"github.com/zeromicro/go-zero/zrpc"
)
type Config struct {
rest.RestConf
GreetRpc zrpc.RpcClientConf
}
根据proto生成代码
syntax = "proto3";
package greet;
option go_package="./greet";
message Request {
string ping = 1;
}
message Response {
string pong = 1;
}
service Greet {
rpc Ping(Request) returns(Response);
}
这个就是rpc的greet.proto
protoc greet.proto --go_out=. --go-grpc_out=.
这里也可用go-zero的, 原理一样, 都是生成
greet.pb.go
和greet_grpc.pb.go
注册到service

package svc
import (
"greet_client/greet"
"greet_client/internal/config"
"github.com/zeromicro/go-zero/zrpc"
)
type ServiceContext struct {
Config config.Config
GreetRpc greet.GreetClient
}
func NewServiceContext(c config.Config) *ServiceContext {
return &ServiceContext{
Config: c,
GreetRpc: greet.NewGreetClient(zrpc.MustNewClient(c.GreetRpc).Conn()),
}
}
写业务代码

package logic
import (
"context"
"greet_client/greet"
"greet_client/internal/svc"
"greet_client/internal/types"
"github.com/zeromicro/go-zero/core/logx"
)
type Greet_clientLogic struct {
logx.Logger
ctx context.Context
svcCtx *svc.ServiceContext
}
func NewGreet_clientLogic(ctx context.Context, svcCtx *svc.ServiceContext) *Greet_clientLogic {
return &Greet_clientLogic{
Logger: logx.WithContext(ctx),
ctx: ctx,
svcCtx: svcCtx,
}
}
func (l *Greet_clientLogic) Greet_client(req *types.Request) (resp *types.Response, err error) {
res, err := l.svcCtx.GreetRpc.Ping(l.ctx, &greet.Request{
Ping: "1236666",
})
if err != nil {
return nil, err
}
return &types.Response{
Message: res.Pong,
}, nil
}